To generate a dataset using tensors in TensorFlow, you can use the tf.data.Dataset.from_tensor_slices() method. This method takes a tensor and creates a dataset with each element being a slice of the tensor along the first dimension. You can then further manipulate the dataset using various methods provided by the tf.data module, such as shuffle, batch, and map. By creating a dataset from tensors, you can efficiently process and feed data into your TensorFlow model during training and evaluation.
Best TensorFlow Books to Read of November 2024
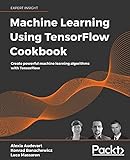
Rating is 5 out of 5
Machine Learning Using TensorFlow Cookbook: Create powerful machine learning algorithms with TensorFlow
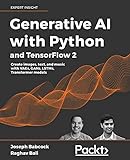
Rating is 4.8 out of 5
Generative AI with Python and TensorFlow 2: Create images, text, and music with VAEs, GANs, LSTMs, Transformer models
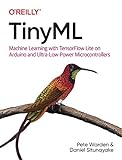
Rating is 4.5 out of 5
TinyML: Machine Learning with TensorFlow Lite on Arduino and Ultra-Low-Power Microcontrollers
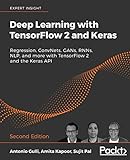
Rating is 4.4 out of 5
Deep Learning with TensorFlow 2 and Keras: Regression, ConvNets, GANs, RNNs, NLP, and more with TensorFlow 2 and the Keras API, 2nd Edition
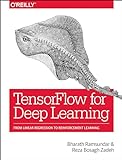
Rating is 4.2 out of 5
TensorFlow for Deep Learning: From Linear Regression to Reinforcement Learning
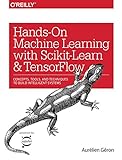
Rating is 4.1 out of 5
Hands-On Machine Learning with Scikit-Learn and TensorFlow: Concepts, Tools, and Techniques to Build Intelligent Systems
How to concatenate tensors in TensorFlow?
In TensorFlow, you can concatenate tensors using the tf.concat()
function. This function concatenates tensors along a specified axis.
Here is an example of how you can concatenate two tensors along the first axis:
1 2 3 4 5 6 7 8 9 10 |
import tensorflow as tf # Create two tensors tensor1 = tf.constant([[1, 2], [3, 4]]) tensor2 = tf.constant([[5, 6]]) # Concatenate the two tensors along the first axis concatenated_tensor = tf.concat([tensor1, tensor2], axis=0) print(concatenated_tensor) |
In this example, the concatenated_tensor
will be [[1, 2], [3, 4], [5, 6]]
.
You can also concatenate tensors along other axes by changing the axis
parameter in the tf.concat()
function.
What is the element-wise multiplication of tensors in TensorFlow?
The element-wise multiplication of tensors in TensorFlow can be achieved using the tf.multiply()
function. This function takes two tensors as input and returns a new tensor with each element being the product of the corresponding elements in the input tensors. For example, if tensor1
and tensor2
are two tensors, we can perform element-wise multiplication as follows:
1 2 3 4 5 6 7 8 |
import tensorflow as tf tensor1 = tf.constant([[1, 2], [3, 4]]) tensor2 = tf.constant([[5, 6], [7, 8]]) result = tf.multiply(tensor1, tensor2) print(result) |
This will output a new tensor containing the element-wise product of tensor1
and tensor2
.
How to convert a numpy array to a tensor in TensorFlow?
You can convert a numpy array to a tensor in TensorFlow using the tf.convert_to_tensor
function. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 |
import tensorflow as tf import numpy as np # Create a numpy array arr = np.array([[1, 2, 3], [4, 5, 6]]) # Convert the numpy array to a tensor tensor = tf.convert_to_tensor(arr) # Print the tensor print(tensor) |
This will output:
1 2 3 |
<tf.Tensor: shape=(2, 3), dtype=int64, numpy= array([[1, 2, 3], [4, 5, 6]])> |
Now, the numpy array arr
has been converted to a TensorFlow tensor tensor
.